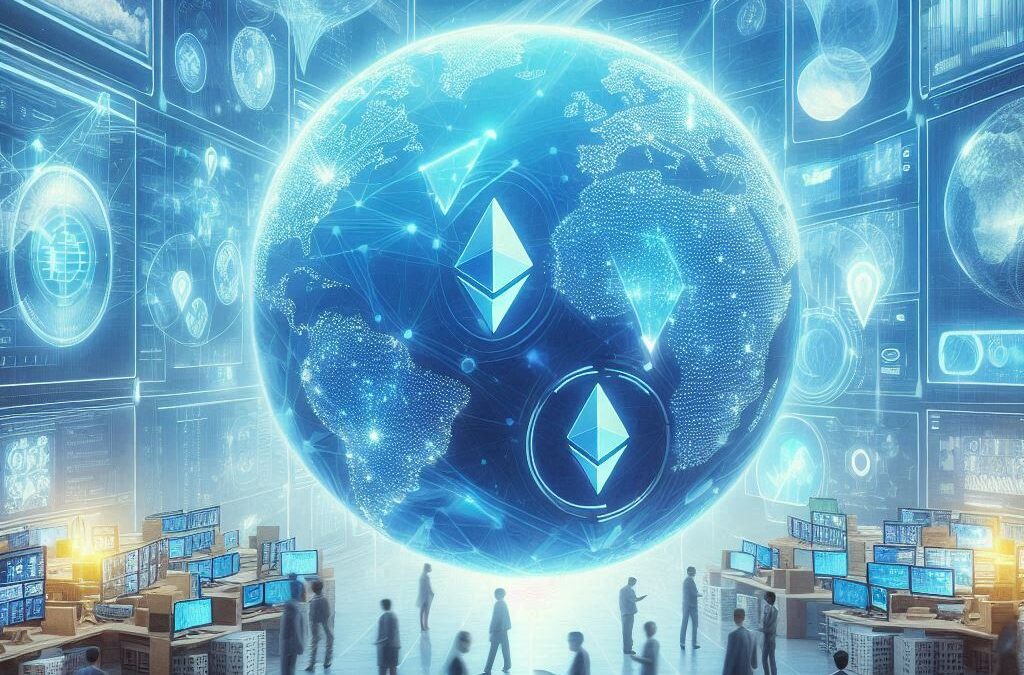
A Guide to Trustless, Peer-to-Peer Commerce
Introduction:
Decentralized marketplaces are transforming how we think about buying, selling, and trading goods and services. Built on blockchain technology, they offer a peer-to-peer (P2P) alternative to traditional, centralized platforms. Ethereum, with its smart contract capabilities, is one of the most popular platforms for building these decentralized systems.
In a decentralized marketplace, there’s no need for intermediaries like eBay or Amazon. Instead, buyers and sellers interact directly, with smart contracts enforcing the rules and ensuring trustless transactions. This guide explores the steps involved in building such a marketplace on Ethereum, using smart contracts to facilitate secure, transparent, and efficient exchanges.
We’ll dive into the essential components, such as how users can list items, handle payments through cryptocurrencies, and manage disputes—all without relying on a central authority. Whether you’re a developer or a blockchain enthusiast, this guide will walk you through the process of implementing your own decentralized marketplace on Ethereum, leveraging the power of decentralization to create a more open and accessible digital economy.
Let’s begin by understanding how decentralized marketplaces work and the architecture behind them.
Why Do We Need Peer-to-Peer (P2P) Commerce?
Peer-to-peer (P2P) commerce addresses several limitations of traditional centralized marketplaces, offering a range of advantages that align with the core values of decentralization and blockchain technology. Here’s why P2P commerce is essential in today’s economy:
- Elimination of Intermediaries
Traditional marketplaces like Amazon or eBay act as intermediaries, charging high fees and controlling the marketplace rules. P2P commerce removes the need for these middlemen, allowing buyers and sellers to interact directly. This results in lower fees, more control over transactions, and a more efficient marketplace. - Trustless Transactions
P2P commerce, especially when built on Ethereum, leverages smart contracts to ensure trustless transactions. Buyers and sellers don’t need to trust each other or rely on a third party to facilitate the deal. Instead, smart contracts automatically enforce the rules, ensuring that the transaction completes only when both parties fulfill their obligations. This reduces fraud, disputes, and delays. - Global Accessibility
Decentralized P2P marketplaces are open to anyone with internet access, removing geographical restrictions and the need for complex payment infrastructure. With cryptocurrency, users can easily trade across borders without dealing with exchange rates or bank transfers, making the marketplace globally accessible to billions of people. - Censorship Resistance
Centralized platforms can block users or restrict certain types of transactions based on political or corporate interests. In contrast, decentralized P2P marketplaces built on Ethereum are inherently censorship-resistant. No single entity can control who participates or what can be traded, fostering a more open and free market. - Increased Security & Privacy
Centralized platforms store vast amounts of user data, making them attractive targets for hackers. P2P marketplaces built on Ethereum provide better security by distributing data across a decentralized network, minimizing the risk of breaches. Furthermore, transactions on blockchain-based P2P marketplaces allow for greater privacy since users don’t need to share sensitive personal information with intermediaries. - Transparency
Every transaction on an Ethereum-based decentralized marketplace is recorded on the blockchain, providing an immutable and transparent record of all activity. This enhances accountability and trust, as buyers and sellers can verify the integrity of each other’s actions by simply reviewing the public ledger. - Ownership and Control
In P2P marketplaces, users retain full ownership and control over their assets. Unlike centralized platforms that can freeze accounts or limit access, decentralized marketplaces empower individuals with self-custody of their digital assets, meaning users are in charge of their funds and personal information at all times. - Innovation and Customization
Decentralized marketplaces encourage innovation. Developers can create unique marketplace features through smart contracts, tailoring them to specific needs, such as automated escrow, reputation systems, and complex multi-party agreements. This level of customization is hard to achieve in traditional marketplaces, which are generally rigid in their design.
In essence, P2P commerce promotes a more efficient, fair, and transparent system for online transactions. By leveraging Ethereum’s blockchain technology, it creates a decentralized marketplace that empowers users with more freedom, control, and security in their trade dealings. The decentralized future of commerce aligns closely with the values of financial inclusivity, user autonomy, and democratized global access.
Implementing a decentralized peer-to-peer (P2P) marketplace on Ethereum involves using Solidity smart contracts to handle various marketplace functions such as item listings, transactions, payments, and dispute resolutions. Below is a breakdown of the key contracts involved in this marketplace and how they work together.
Key Contracts:
- Marketplace Contract: The main contract that manages listings, transactions, and payments.
- Escrow Contract: Holds payments in escrow until the transaction is complete.
- Reputation System Contract: Manages user ratings and reputation based on transaction outcomes.
- Dispute Resolution Contract: Facilitates resolving disputes between buyers and sellers.
1. Marketplace Contract
This contract allows users to list items, buy items, and interact with the marketplace.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "./Escrow.sol";
import "./ReputationSystem.sol";
contract Marketplace {
struct Listing {
uint id;
address seller;
string item;
uint price;
bool sold;
}
uint public listingCounter = 0;
mapping(uint => Listing) public listings;
Escrow public escrow;
ReputationSystem public reputationSystem;
event Listed(uint indexed listingId, address indexed seller, string item, uint price);
event Purchased(uint indexed listingId, address indexed buyer, address indexed seller, uint price);
constructor(address escrowAddress, address reputationSystemAddress) {
escrow = Escrow(escrowAddress);
reputationSystem = ReputationSystem(reputationSystemAddress);
}
// Function to list an item for sale
function listItem(string memory _item, uint _price) external {
listingCounter++;
listings[listingCounter] = Listing(listingCounter, msg.sender, _item, _price, false);
emit Listed(listingCounter, msg.sender, _item, _price);
}
// Function to purchase an item
function buyItem(uint _listingId) external payable {
Listing storage listing = listings[_listingId];
require(msg.value == listing.price, "Incorrect price sent.");
require(!listing.sold, "Item already sold.");
// Create escrow for the transaction
escrow.createEscrow{value: msg.value}(msg.sender, listing.seller, listing.price, _listingId);
listing.sold = true;
emit Purchased(_listingId, msg.sender, listing.seller, listing.price);
}
// Mark transaction as complete (called by the escrow)
function completeTransaction(uint _listingId) external {
Listing storage listing = listings[_listingId];
require(listing.sold, "Item not sold yet.");
reputationSystem.updateReputation(listing.seller, true);
reputationSystem.updateReputation(msg.sender, true);
}
// Function to handle disputes (calling the dispute resolution)
function resolveDispute(uint _listingId, address _winner) external {
Listing storage listing = listings[_listingId];
require(listing.sold, "Item not sold yet.");
// Resolve dispute and update reputation accordingly
reputationSystem.updateReputation(_winner, true);
reputationSystem.updateReputation(listing.seller == _winner ? listing.seller : msg.sender, false);
}
}
2. Escrow Contract
This contract holds payments in escrow until the transaction is completed.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract Escrow {
struct EscrowDetails {
address buyer;
address seller;
uint amount;
bool isCompleted;
}
mapping(uint => EscrowDetails) public escrows;
uint public escrowCounter = 0;
event EscrowCreated(uint indexed escrowId, address indexed buyer, address indexed seller, uint amount);
event EscrowReleased(uint indexed escrowId);
// Function to create an escrow
function createEscrow(address _buyer, address _seller, uint _amount, uint _listingId) external payable {
require(msg.value == _amount, "Incorrect amount sent to escrow.");
escrowCounter++;
escrows[escrowCounter] = EscrowDetails(_buyer, _seller, _amount, false);
emit EscrowCreated(escrowCounter, _buyer, _seller, _amount);
}
// Function to release the funds to the seller
function releaseFunds(uint _escrowId) external {
EscrowDetails storage escrow = escrows[_escrowId];
require(!escrow.isCompleted, "Escrow already completed.");
escrow.isCompleted = true;
payable(escrow.seller).transfer(escrow.amount);
emit EscrowReleased(_escrowId);
}
// Function to handle dispute and release funds to winner
function resolveDispute(uint _escrowId, address _winner) external {
EscrowDetails storage escrow = escrows[_escrowId];
require(!escrow.isCompleted, "Escrow already completed.");
escrow.isCompleted = true;
payable(_winner).transfer(escrow.amount);
emit EscrowReleased(_escrowId);
}
}
3. Reputation System Contract
This contract manages reputation for buyers and sellers based on the outcome of transactions.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract ReputationSystem {
mapping(address => uint) public reputations;
event ReputationUpdated(address indexed user, uint newReputation);
// Function to update reputation
function updateReputation(address _user, bool _success) external {
if (_success) {
reputations[_user] += 1; // Increase reputation on successful transaction
} else {
reputations[_user] = reputations[_user] > 0 ? reputations[_user] - 1 : 0; // Decrease on failure
}
emit ReputationUpdated(_user, reputations[_user]);
}
// Function to get the reputation of a user
function getReputation(address _user) external view returns (uint) {
return reputations[_user];
}
}
4. Dispute Resolution Contract
This contract could be used to resolve disputes between buyers and sellers. In this simple implementation, we assume that an off-chain arbitrator decides who wins.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "./Escrow.sol";
contract DisputeResolution {
Escrow public escrow;
event DisputeResolved(uint indexed escrowId, address winner);
constructor(address escrowAddress) {
escrow = Escrow(escrowAddress);
}
// Function to resolve disputes and release funds to the winner
function resolveDispute(uint _escrowId, address _winner) external {
escrow.resolveDispute(_escrowId, _winner);
emit DisputeResolved(_escrowId, _winner);
}
}
How These Contracts Work Together:
- Item Listing: The seller lists an item for sale using the
Marketplace
contract. - Purchasing the Item: A buyer sends the exact amount to the
Marketplace
contract, which then creates an escrow using theEscrow
contract to hold the payment. - Completing the Transaction: Once the buyer receives the item, they confirm the purchase, and the
Escrow
contract releases the funds to the seller. - Reputation Update: After the transaction, the
Marketplace
contract calls theReputationSystem
contract to update the reputation of both the buyer and the seller. - Dispute Resolution: If there’s a dispute, the
DisputeResolution
contract resolves it by deciding who should receive the funds, interacting with theEscrow
contract.
This setup ensures a trustless, transparent P2P marketplace by leveraging smart contracts to manage transactions, escrow, and disputes.
Conclusion:
Implementing a decentralized peer-to-peer marketplace on Ethereum empowers users with a secure, trustless, and transparent platform for commerce. By leveraging smart contracts, key functionalities such as item listings, escrow services, reputation management, and dispute resolution are handled autonomously without intermediaries. This architecture ensures that both buyers and sellers have full control over their transactions, reducing fees and fostering global accessibility.
The combination of Ethereum’s blockchain, smart contracts, and a decentralized structure makes these marketplaces censorship-resistant, secure, and open to innovation. As blockchain adoption continues to grow, decentralized marketplaces will play a crucial role in reshaping digital commerce, providing a more democratic and equitable system for users worldwide.
Through this guide, we’ve seen how various smart contracts interact to ensure seamless and secure operations in a decentralized marketplace. The future of commerce lies in decentralization, and Ethereum offers the perfect platform to build that future.