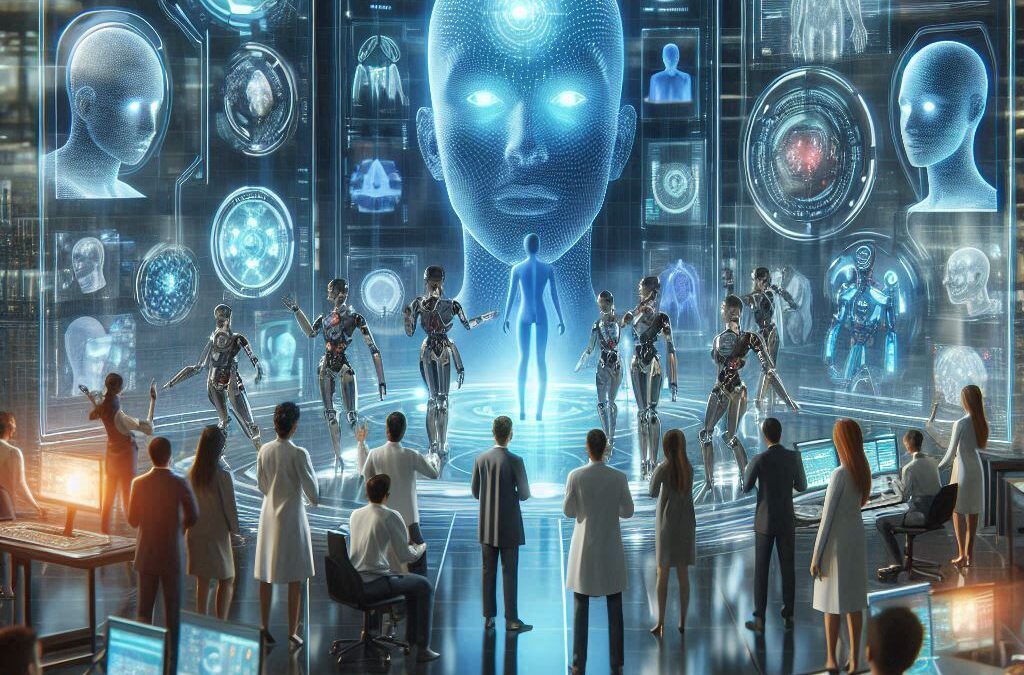
Build Your First Multi-Agent System with CrewAI: A Real-Time Example
Introduction
Multi-agent systems (MAS) are gaining significant attention in the AI landscape, enabling collaboration between autonomous agents to accomplish complex tasks efficiently. CrewAI is one of the leading frameworks designed to simplify multi-agent orchestration, allowing developers to build intelligent, cooperative AI agents. In this post, we will walk through building a multi-agent system using CrewAI with a real-time example: an AI-powered Resume Optimizer that matches a candidate’s resume with a given job description.
What is CrewAI?
CrewAI is a powerful framework that enables the creation of AI agents that can collaborate, communicate, and execute tasks efficiently. It provides built-in capabilities to:
- Define different agents with distinct roles and skills.
- Assign tasks to agents based on their expertise.
- Enable agents to communicate and refine results collaboratively.
Real-Time Example: Resume Optimizer
Problem Statement
Job seekers often struggle to tailor their resumes to match specific job descriptions. An optimized resume increases the chances of passing through automated applicant tracking systems (ATS) and getting shortlisted for interviews. We will build a Resume Optimizer where AI agents work together to refine a candidate’s resume based on a job description.
Multi-Agent System Design
For this system, we will define the following agents:
- Resume Parser Agent – Extracts key sections (experience, skills, education) from the candidate’s resume.
- Job Description Analyzer Agent – Extracts required skills, experience, and keywords from the job description.
- Match & Gap Analysis Agent – Compares the extracted resume data with job requirements and identifies missing or weak areas.
- Resume Enhancement Agent – Suggests modifications to improve keyword relevance and alignment with the job.
- Final Review Agent – Performs a final quality check and generates the optimized resume.
Implementing the Multi-Agent System with CrewAI
Step 1: Install CrewAI
Ensure you have CrewAI installed. If not, install it using pip:
pip install crewai
Step 2: Define the Agents
Each agent is responsible for a specific task. We define them using CrewAI’s API:
from crewai import Agent
resume_parser = Agent(
name="Resume Parser",
role="Extracts key sections from the resume",
goal="Identify work experience, skills, education, and achievements",
backstory="An AI expert trained in NLP to parse resumes and extract structured information."
)
job_analyzer = Agent(
name="Job Description Analyzer",
role="Analyzes job descriptions to extract required skills and experience",
goal="Identify key skills, experience requirements, and relevant keywords",
backstory="An AI trained in text analytics to extract crucial hiring manager expectations."
)
match_analyzer = Agent(
name="Match & Gap Analysis",
role="Compares resume with job description",
goal="Identify missing skills and suggest improvements",
backstory="An AI specializing in resume screening and ATS optimization."
)
Step 3: Define the Tasks
We now define tasks for each agent:
from crewai import Task
parse_resume_task = Task(
description="Extract key information (work experience, skills, education) from the provided resume.",
expected_output="Structured JSON with extracted resume details",
agent=resume_parser
)
analyze_job_task = Task(
description="Extract required skills and experience from the job description.",
expected_output="Structured JSON with job requirements",
agent=job_analyzer
)
match_gap_task = Task(
description="Compare the extracted resume details with job requirements and highlight gaps.",
expected_output="List of missing skills and suggested improvements",
agent=match_analyzer
)
Step 4: Execute the Workflow
Finally, we create a Crew to orchestrate the workflow:
from crewai import Crew
resume_optimizer_crew = Crew(
agents=[resume_parser, job_analyzer, match_analyzer],
tasks=[parse_resume_task, analyze_job_task, match_gap_task]
)
result = resume_optimizer_crew.kickoff()
print(result)
Conclusion
By leveraging CrewAI, we built a simple yet powerful Resume Optimizer multi-agent system. This approach can be extended to various real-world applications, such as automated research assistants, customer support AI teams, or content generation workflows.
Next Steps
- Experiment with adding more agents (e.g., a Cover Letter Generator Agent).
- Enhance agents with more sophisticated NLP models like GPT-4 or BERT.
- Deploy the system as an API for users to optimize resumes on demand.
CrewAI makes it incredibly easy to design and deploy multi-agent AI solutions. Start building today and explore the power of AI collaboration!